protostuff序列化
引言
HTTP通信离不开对象的序列化和反序列化。通过序列化技术,可以跨语言实现数据的传输,将对象转换为字节序列,然后在网络上传送;通过反序列化,可以将字节序列转换为对象。 基本原理和网络通信是一致的,通过特殊的编码方式,写入数据将对象以及其内部数据编码,存在在数组或者文件里面然后发送到目的地后,在进行解码,读出数据。
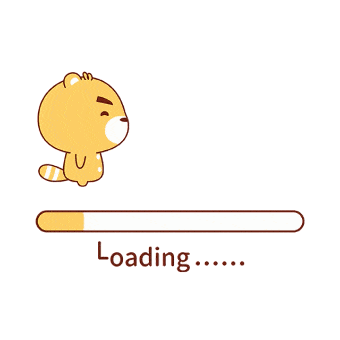
protostuff
protostuff是Google出品的一种轻量并且高效的结构化数据存储格式,性能比 JSON
、XML
要高很多。
之所以性能如此好,主要得益于两个:第一,它使用 proto 编译器,自动进行序列化和反序列化,速度非常快,应该比 XML
和 JSON
快上了 20~100
倍;第二,它的数据压缩效果好,就是说它序列化后的数据量体积小。因为体积小,传输起来带宽和速度上会有优化。
详细效率对比可参考:java序列化/反序列化之xstream、protobuf、protostuff 的比较与使用例子
maven依赖
1 | <dependency> |
继承父类方式
1 | public interface CanProto { |
1 | public class ProtoBase implements CanProto, Serializable { |
此方式可以使用Javabean继承ProtoBase类实现序列化。
ProtostuffUtil工具类方式
ProtostuffUtil.java
1 | import io.protostuff.LinkedBuffer; |
测试bean
Student.java
1 | import io.protostuff.Tag; |
test类
ProtostuffUtilTest.java
1 | import java.util.Arrays; |
输出
1 | serializer result:[10, 12, 114, 97, 105, 110, 98, 111, 119, 104, 111, 114, 115, 101, 18, 11, 50, 48, 49, 49, 50, 50, 49, 52, 48, 49, 48, 24, 24, 34, 6, 90, 78, 77, 90, 68, 88, 40, 0] |
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 彩虹马的博客!
评论